Bootstrap 4 offers carousel integration with slide and fade effects. We can turn the Bootstrap 4 carousel in a full-screen slider with help of available classes and custom rules. Mostly you can see them as background image responsive sliders that cover the entire viewport.
Here, I will explain you steps to spread a Bootstrap 4 carousel to a full height and width slider. Also, you can see one such carousel on our corporate website. Further, I have provided PHP code to produce slider markup. You can create the carousel within minutes by copy-pasting and just providing right values.
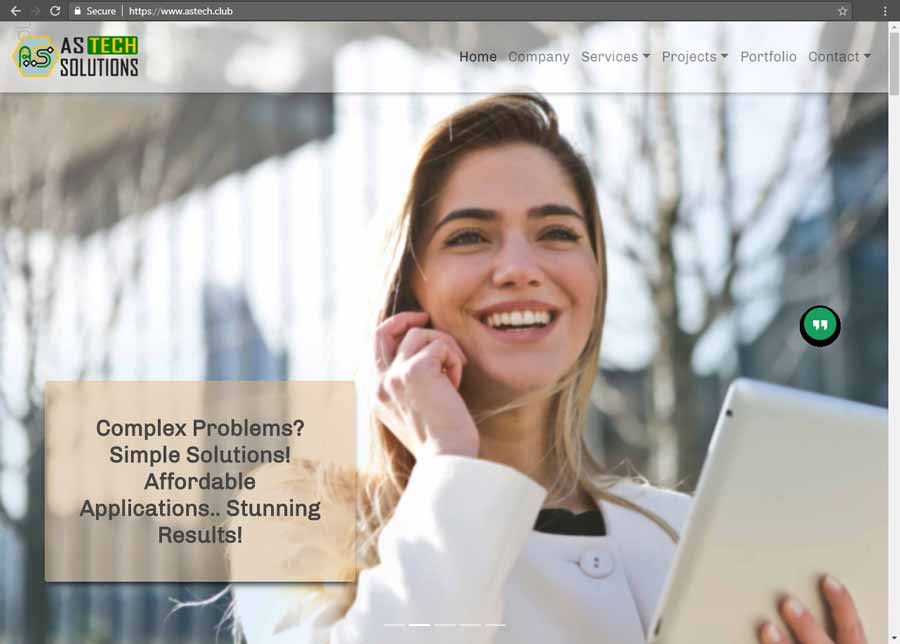
Bootstrap 4 Carousel Full-screen Slider
1 2 3 4 5 6 7 8 9 10 | <div id="bs_fs_caro" class="carousel slide full-screen-carousel" data-ride="carousel"> <div class="carousel-inner h-100"> <div class="carousel-item active h-100"> <!--Slide content--> </div> <div class="carousel-item h-100"> <!--Slide content--> </div> </div> </div> |
The above is the fundamental markup of the carousel in Bootstrap 4 with full height coverage. Further, you have noticed a few additional classes extra there. First, the outmost carousel container needs to cover full viewport height. So here the full-screen-carousel
class comes into play. The CSS rule below does the job.
1 2 3 4 | .full-screen-carousel { height: 100vh; /*margin-top: -100px;*/ } |
Your container might have some distance from the top of the page and you may want to remove that. In such case, use negative margin trick. Next, inner carousel div, as well as each carousel div in that have h-100 class. The h-100 utility class is already provided in Bootstrap 4. The class make the container as tall as it’s parent.
Finally, put content for each slide item and a full-screen Bootstrap 4 carousel is ready. The content might be anything among background images and captions with headings/subheadings.
Convert Bootstrap 4 single column dropdown to a multi-column navigation menu. Or have Bootstrap + WordPress pagination.
PHP Background Image Slider using Bootstrap 4 Carousel
PHP has made programming life a lot easier. Many of you might wish to implement the same full-screen Bootstrap carousel in PHP. Below is the flexible and extensible script and supports background image sliding as well.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 | <?php $s_id = 'bs_fs_caro'; //Slider Id $fade = 1; //Fade transition instead of slide $active = 0; //Slide number to start carousel from, zero based indexing $show_arrows = 1; //Show previous/next arrows, 1 => show, 0 => don't show $show_indicators = 1; //Show indicators, 1 => show, 0 => don't show $img_path = 'http://www.yourwebsite.com/images/'; //URL to the directory containing background images $slides = array( //Slides array( 'bg' => 'awesome.jpg', 'title' => 'You Are Awesome!', 'subtitle' => '"Awesome, awesome, awesome!" she exclaimed, and clapped her hands.' ), array( 'bg' => 'fantastic.jpg', 'title' => 'You Are Fantastic!', 'subtitle' => 'Many of the emendations suggested are more fantastic than felicitous.' ), array( 'bg' => 'marvellous.jpg', 'title' => 'You Are Marvellous!', 'subtitle' => 'That\'s a marvellous description, Mrs Drummond. You\'re unusually observant.' ), array( 'bg' => 'splendid.jpg', 'title' => 'You Are Splendid!', 'subtitle' => 'What a splendid reign the Emperor Alexander\'s might have been!' ), ); if($slides) : $content = array(); foreach($slides as $k => $v) //Content within each slide $content[] = sprintf('<h2 class="text-primary pb-5">%s</h2><h4 class="text-secondary">%s</h4>', $v['title'], $v['subtitle']); $active = isset($slides[$active]) ? $active : 0; ?> <!--Carousel start--> <div id="<?php echo $s_id; ?>" class="carousel slide full-screen-carousel<?php echo($fade ? ' carousel-fade' : ''); ?>" data-ride="carousel"> <?php if($show_indicators) { //Carousel indicators ?> <ol class="carousel-indicators"> <?php foreach($slides as $k => $v) { ?> <li data-target="#<?php echo $s_id; ?>" data-slide-to="<?php echo $k; ?>" class="<?php echo ($k == $active ? 'active' : ''); ?>"></li> <?php } ?> </ol> <?php } //Carousel indicators end ?> <div class="carousel-inner h-100"> <?php foreach($slides as $k => $v) { ?> <div class="carousel-item h-100 bgfs<?php echo ($k == $active ? ' active' : ''); ?>" style="background-image: url(<?php echo $img_path.$v['bg']; ?>);"> <div class="carousel-caption bsfs-caption"><?php echo $content[$k]; ?></div> </div> <?php } ?> </div> <?php if($show_arrows) { //Carousel arrows ?> <a class="carousel-control-prev" href="#<?php echo $s_id; ?>" role="button" data-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="sr-only">Previous</span> </a> <a class="carousel-control-next" href="#<?php echo $s_id; ?>" role="button" data-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="sr-only">Next</span> </a> <?php } //Carousel arrows end ?> </div> <!--Carousel End--> <?php endif; ?> |
I assume that you have already included the required Bootstrap CSS and JS files in your project. You need to configure as well as supply values to highlighted parameters. First, from line 2 to 7, you can see the purpose of each variable in the respective comment.
Lines 8 to 29 contain data for each slide. The array keys and values both depend on what do you want to show in the carousel. Notice the line #35, I need a title and a subtitle. Additionally, the line #49 uses the background image.
You can change the $content
variable as per your wish. But remember to change $slides
array accordingly. You don’t require to change anything between lines 38-65 unless you wish to customize Bootstrap 4 carousel highly. Similarly, remove the style element from line #49 to remove background images in slides.
So it’s the PHP code that creates a full-flagged Bootstrap 4 carousel as a full-screen background images slider. But wait, we need a bit of CSS as well. Duh!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <style> .full-screen-carousel { height: 100vh; /*margin-top: -100px;*/ } .bgfs { background-color: #ccc; background-size: cover; background-position: center; } .bsfs-caption { top: 30%; bottom: auto; } </style> |
You need to place these CSS rules after the Bootstrap CSS. The first one makes the carousels to cover full height. Likewise same, the second rule spreads the background to the entire viewport. And the last rule shifts the Bootstrap 4 carousel-caption class to 30% below from the top. By default, this class places the caption 20px above the bottom.
Here you have understood how can you turn a BS4 carousel to full-width and height. Further, the h-100 utility class made it easier as compared to version 3. You completed creating a Bootstrap 4 carousel full-screen background slider in PHP.
You want to customize it more or have a question? Want to use in a WordPress theme? Write to us using the comment form given.