You just need a function to add Custom Taxonomies to Custom Post Types. In the previous article, we made WordPress default taxonomies called Category & Tag to support our CPTs. Here we are going to create custom taxonomies for those CPTs.
In earlier two articles of CPTs series, we learnt to create Custom Post Types and used WP categories & tags taxonomies in them. This article is 3rd continuous part of CPTs series:
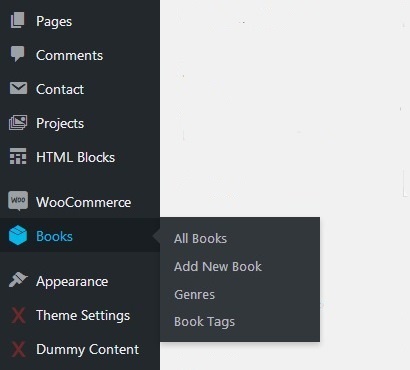
Custom taxonomies for custom post type content
Add Custom Taxonomies to Custom Post Types
In your functions.php or plugin specific file, write the following function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | function my_cpt_taxonomies() { // Add new taxonomy, make it hierarchical (like categories) $labels = array( 'name' => _x( 'Genres', 'taxonomy general name', 'text_domain' ), 'singular_name' => _x( 'Genre', 'taxonomy singular name', 'text_domain' ), 'search_items' => __( 'Search Genres', 'text_domain' ), 'all_items' => __( 'All Genres', 'text_domain' ), 'parent_item' => __( 'Parent Genre', 'text_domain' ), 'parent_item_colon' => __( 'Parent Genre:', 'text_domain' ), 'edit_item' => __( 'Edit Genre', 'text_domain' ), 'update_item' => __( 'Update Genre', 'text_domain' ), 'add_new_item' => __( 'Add New Genre', 'text_domain' ), 'new_item_name' => __( 'New Genre Name', 'text_domain' ), 'menu_name' => __( 'Genre', 'text_domain' ), ); $args = array( 'hierarchical' => true, 'labels' => $labels, 'show_ui' => true, 'show_admin_column' => true, 'query_var' => true, 'rewrite' => array( 'slug' => 'genre', 'with_front' => false ), ); register_taxonomy( 'genre', array( 'book' ), $args ); // Add new taxonomy, NOT hierarchical (like tags) $labels = array( 'name' => _x( 'Book Tags', 'taxonomy general name', 'text_domain' ), 'singular_name' => _x( 'Book Tag', 'taxonomy singular name', 'text_domain' ), 'search_items' => __( 'Search Book Tags', 'text_domain' ), 'popular_items' => __( 'Popular Book Tags', 'text_domain' ), 'all_items' => __( 'All Book Tags', 'text_domain' ), 'parent_item' => null, 'parent_item_colon' => null, 'edit_item' => __( 'Edit Book Tag', 'text_domain' ), 'update_item' => __( 'Update Book Tag', 'text_domain' ), 'add_new_item' => __( 'Add New Book Tag', 'text_domain' ), 'new_item_name' => __( 'New Book Tag', 'text_domain' ), 'separate_items_with_commas' => __( 'Separate book tags with commas', 'text_domain' ), 'add_or_remove_items' => __( 'Add or remove book tags', 'text_domain' ), 'choose_from_most_used' => __( 'Choose from the most used book tags', 'text_domain' ), 'not_found' => __( 'No book tag found.', 'text_domain' ), 'menu_name' => __( 'Tags', 'text_domain' ), ); $args = array( 'hierarchical' => false, 'labels' => $labels, 'show_ui' => true, 'show_admin_column' => true, 'update_count_callback' => '_update_post_term_count', 'query_var' => true, 'rewrite' => array( 'slug' => 'book_tag' ), ); register_taxonomy( 'book_tag', 'book', $args ); } |
Function register_taxonomy()
The register_taxonomy() function does all the things for you. It has a number of parameters to pass which you should read for better understanding.
Here we are creating two taxonomies for book post type. First is Genre which is a hierarchical taxonomy that follows parent-child relationship and is same as Category for Post post type. Another is Book Tag which is multifaceted (non-hierarchical) taxonomy.
Parameters:
show_ui
: Pass true to generate a default UI for managing this taxonomy.show_admin_column
: This is used to enable automatic creation of taxonomy columns on the associated post-types table.query_var
: Allows uses of default WP_Query if true passed.with_front
: We don’t want to display category base before taxonomy slug. If fiction is a term created in genre taxonomy, the URL slug will be:/genre/fiction/update_count_callback
: To ensure that the custom taxonomy behaves like a tag, you must add the option ‘update_count_callback’ => ‘_update_post_term_count’.
The first argument passed to register_taxonomy() function is the name of the taxonomy object. Please note that WordPress has a lot reserved terms which can be seen when reading about the function. Don’t use any reserved term while providing slug or arguments to add custom taxonomies.
The second argument is array/string with the name of custom post type object. You can add more post types here including default WordPress post types too.
Hook into init to Add Custom Taxonomies
The function we created above needs to hook into init action. check our initial my_posttype_and_taxonomy()
function where we have commented out call to my_cpt_taxonomies()
at line no #34. Remove comment syntax (two trailing slashes) from there and you’re done.
Refresh Permalink to Create Custom Taxonomy Slug
Before you could start working with custom taxonomies or can add custom fields for these taxonomies, you need to refresh permalink to let them work perfectly. Go to Settings -> Permalinks from dashboard menus and just click Save changes button once. Now check if Genres and Book Tags sub-menus are there under Books menu.
So here is all the way to create or add custom taxonomies for custom post types in WordPress. In next article, we will see how to create custom fields for these taxonomies/ CPTs and show them is the dashboard.
Read next: Add Custom Fields to CPT.