By default an opened modal popup window gets close whenever an user presses ESC key on keyboard. In some cases we need to prevent popup window from getting close by escape key as a part of our requirements. Here I have written two methods to disable the function of ESC key using JavaScript and jQuery each.
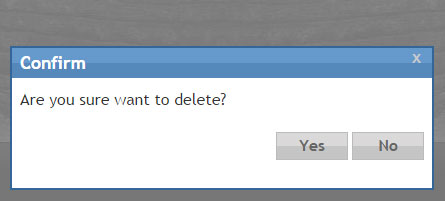
Stop ESC key from closing popup window using JavaScript:
The simplest method using JavaScript is
1 2 3 4 5 | window.onkeydown = function(e){ if(e.keyCode === 27){ // Key code for ESC key e.preventDefault(); } }; |
Prevent popup window from getting close by escape key using jQuery:
You can achieve the same using jQuery
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | $(document).keydown(function(e) { // ESCAPE key pressed if (e.keyCode == 27) { return false; } }); //OR $("body").on("keyup", function(e){ if (e.which === 27){ return false; } }); |
This code above will disable the escape key from closing and this might interfere with other functions, and you probably could specify what element you want the event attached to.
Please note: You should place the code at the end of body to take precedence since most of the time there would be some code already attached in third party scripts which acts default behaviour (closing modal popup on ESC pressing) like the code below:
1 2 3 4 5 | $('body').keyup(function(e) { if(e.which === 27){ $(modal).trigger('reveal:close'); } }); |
Thank you