Here I am writing minimum code to make Top Fixed Menu Bar on Scroll with CSS & jQuery. The purpose of this article is to have the navigation menu fixed at top when visitors scroll down the page and place menu back to it’s original position when scrolling back to upside.
There are plenty of tutorials on internet but they contain lots of script and CSS to obtain the fixing as well as there is a little buggy behaviour: The page jumps up a little when navigation becomes on the top of everything while using those tutorials.
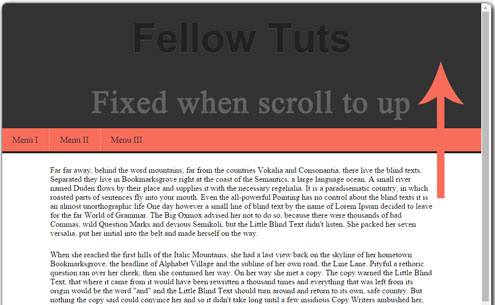
In this article we are nicely creating Top Fixed Menu Bar on Scroll with CSS & jQuery with less code required (minimum JS and CSS) and no page jumping, only smooth scrolling!
jQuery Library
We need jQuery library in our page so download from jQuery site and add it or you can use other jQuery libraries from CDN as well.
1 | <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js" type="text/javascript"></script> |
HTML
The HTML contains our menus made using unordered list. If you already have that then use them and don’t forget to replace the classes in JS code.
1 2 3 4 5 6 7 8 9 | <div class="nav-container"> <div class="nav"> <ul> <li><a href="">Menu I</a></li> <li><a href="">Menu II</a></li> <li><a href="">Menu III</a></li> </ul> </div> </div> |
CSS
The two required CSS rules to apply when menu reaches at top are:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | .fix-nav { /* this make our menu fixed top */ z-index: 9999; position: fixed; left: 0; top: 0; width: 100%; } .fix-body { /* this will adjust body top margin to prevent content jumping when nav gets fix */ margin-top: 50px; /* adjust as per height of your menu */; } |
The first rule has z-index
very high as we don’t want any other absolute element to be on top of our navigation. The second rule set the margin-top
of the body (offset) to the height of the menu when you fix the menu, this stops the rest of the content “jumping” by the height of the menu when it becomes fixed.
Rest of the CSS rules are used for formatting and horizontal appearance of the menu:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | .nav-container { background-color: #f86d5a; } .nav { /* total height of menu is 50px */ height: 46px; border-bottom: 4px solid #222; } .nav ul { list-style: none; } .nav ul li { float: left; line-height: 46px; } .nav ul li a { } .nav ul li a:hover { } |
Javascript
This small JS code below contains function to obtain top fixed menu bar on scroll:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | <script type="text/javascript"> jQuery("document").ready(function($){ var nav = $('.nav-container'); var pos = nav.offset().top; $(window).scroll(function () { var fix = ($(this).scrollTop() > pos) ? true : false; nav.toggleClass("fix-nav", fix); $('body').toggleClass("fix-body", fix); } ); } ); </script> |
This code adds/removes required classes to corresponding elements depending upon current scroll position and top offset coordinate of the nav elements wrapper (nav-container
) using jQuery toggleClass
. This behavior is similar to:
1 2 3 4 5 6 7 8 9 | $(window).scroll(function () { if ($(this).scrollTop() > 250) { $('.nav-container').addClass("fix-nav"); $('body').addClass("fix-body"); } else { $('.nav-container').removeClass("fix-nav"); $('body').removeClass("fix-body"); } }); |
That’s all and it’s easy to implement. VIEW DEMO or the complete code is here!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 | <html> <head> <title> Complete code to create fixed menu on scroll </title> <style type="text/css"> * { margin: 0; padding: 0; } h1 { background-color: #333; color: #222; font: bold 80px "arial"; height: 200px; padding: 25px; text-align: center; text-shadow: 0 1px 1px #4d4d4d; } p { margin-top: 25px; } .nav-container { background-color: #f86d5a; } .nav { height: 46px; border-bottom: 4px solid #222; } .nav ul { list-style: none; } .nav ul li { float: left; line-height: 46px; border-right: 2px solid #f56956; border-left: 2px solid #f9897a; } .nav ul li:first-child { border-left: none; } .nav ul li:last-child { border-right: none; } .nav ul li a { display: block; text-decoration: none; color: #333; font-size: 1.1em; padding-left: 20px; padding-right: 20px; } .nav ul li a:hover { background-color: #222; color: #f86d5a; } .fix-nav { /* this make our menu fixed top */ z-index: 9999; position: fixed; left: 0; top: 0; width: 100%; } .fix-body { /* this will adjust body top margin to prevent content jumping when nav gets fix */ margin-top: 50px; } .content { width: 80%; margin: 25px auto; } </style> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js" type="text/javascript"> </script> <script type="text/javascript"> jQuery("document").ready(function($){ var nav = $('.nav-container'); var pos = nav.offset().top; $(window).scroll(function () { var fix = ($(this).scrollTop() > pos) ? true : false; nav.toggleClass("fix-nav", fix); $('body').toggleClass("fix-body", fix); } ); } ); </script> </head> <body> <div> <h1> Fellow Tuts </h1> </div> <div class="nav-container"> <div class="nav"> <ul> <li> <a href=""> Menu I </a> </li> <li> <a href=""> Menu II </a> </li> <li> <a href=""> Menu III </a> </li> </ul> </div> </div> <div class="content"> <p> Far far away, behind the word mountains, far from the countries Vokalia and Consonantia, there live the blind texts. Separated they live in Bookmarksgrove right at the coast of the Semantics, a large language ocean. A small river named Duden flows by their place and supplies it with the necessary regelialia. It is a paradisematic country, in which roasted parts of sentences fly into your mouth. Even the all-powerful Pointing has no control about the blind texts it is an almost unorthographic life One day however a small line of blind text by the name of Lorem Ipsum decided to leave for the far World of Grammar. The Big Oxmox advised her not to do so, because there were thousands of bad Commas, wild Question Marks and devious Semikoli, but the Little Blind Text didn't listen. She packed her seven versalia, put her initial into the belt and made herself on the way. </p> <p> put more content... </p> </div> </body> </html> |